Hi, I'm trying to implement cubemap textures for my skybox. So far I understand that this requires a set of UVW coordinates from the cube model to be passed into the vertex shader. However, the cube model I am using did not have a set of three coordinates, only two. So I added the third coordinate to the model file, and this mapped the cubemap across the skybox, except it looks like this (the red lines are the markings on the cubemap for identifying the face):
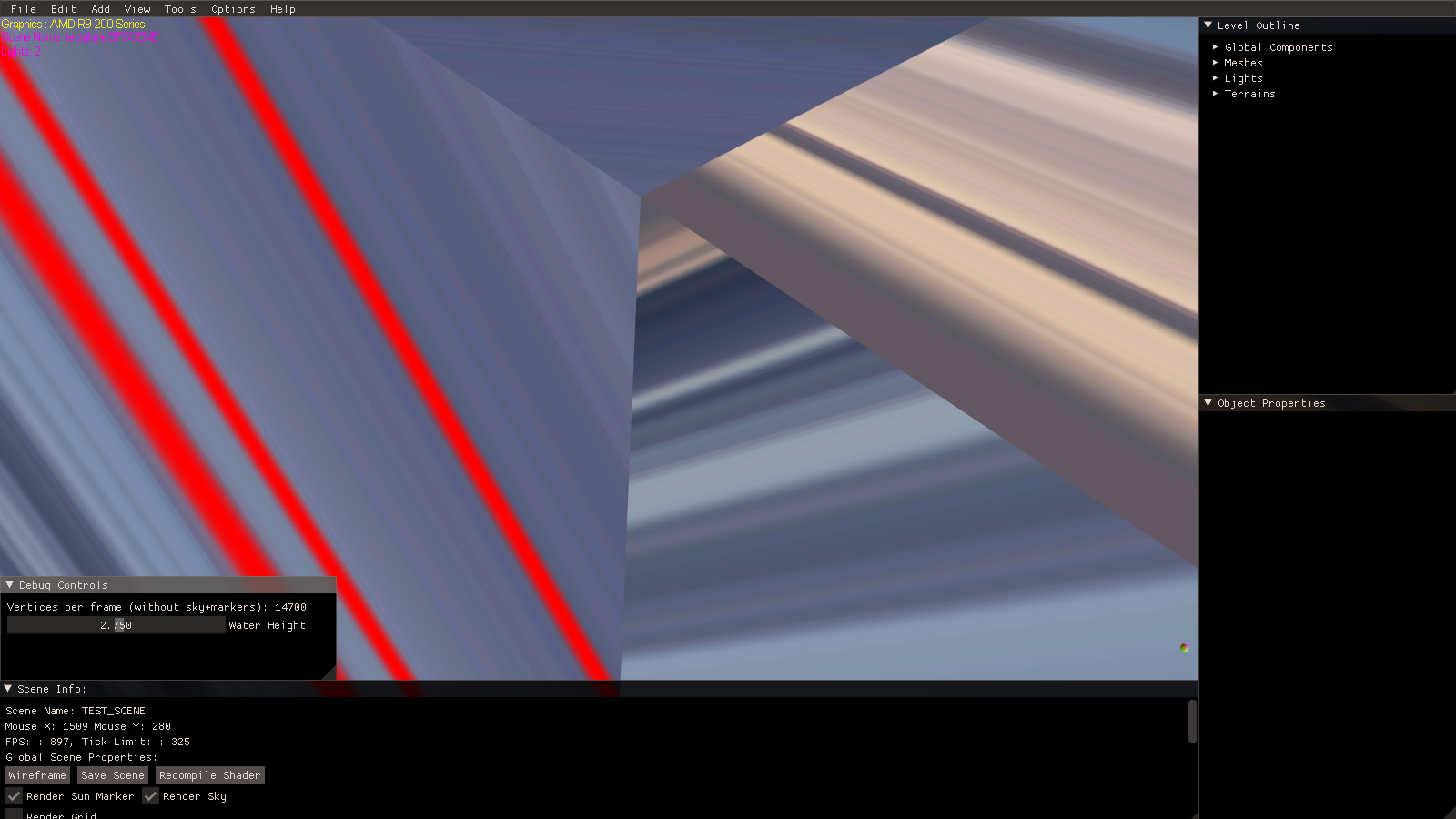
As you can see the texture is warped, this is because all of the W texture coordinates are currently 0.0. But how can I figure out the correct UVW coordinates when using a cubemap? Is there a way to automatically calculate it, or do I have to figure it out myself, and if so what is the method behind this?
This is how the cubemap looks in photoshop, I save it as .DDS with the ‘cubemap’ option:
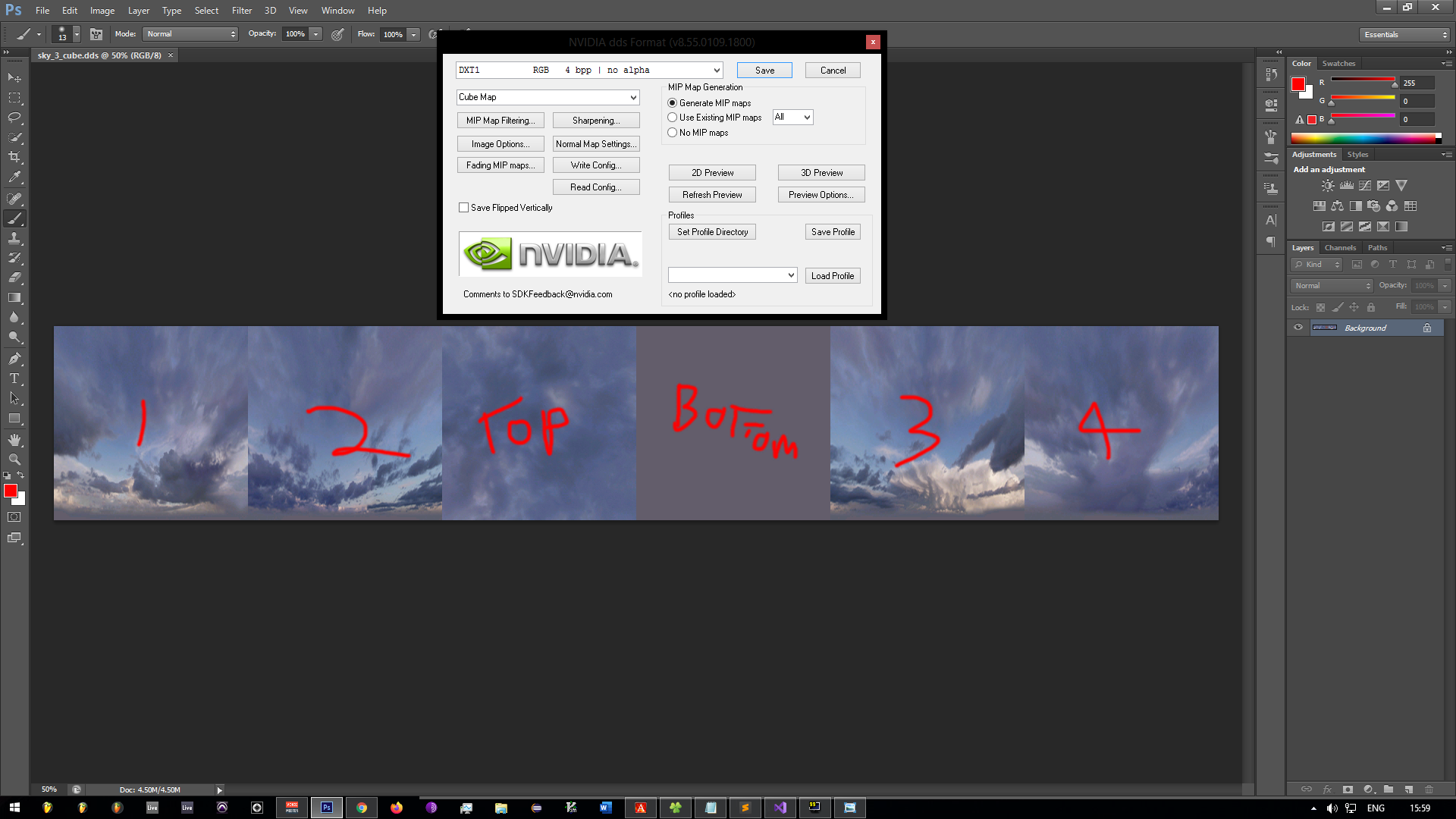
This is how I load the cubemap as a texture:
D3DX11_IMAGE_LOAD_INFO loadSMInfo;
loadSMInfo.MiscFlags = D3D11_RESOURCE_MISC_TEXTURECUBE;
ID3D11Texture2D* SMTexture = 0;
result = D3DX11CreateTextureFromFile(device,filename,
&loadSMInfo, 0, (ID3D11Resource**)&SMTexture, 0);
if (FAILED(result)) {
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(hConsole, 12);
std::cout << "CUBEMAPERROR: TEXTURE PATH NOT FOUND " << result << std::endl;
SetConsoleTextAttribute(hConsole, 15);
return false;
}
D3D11_TEXTURE2D_DESC SMTextureDesc;
SMTexture->GetDesc(&SMTextureDesc);
D3D11_SHADER_RESOURCE_VIEW_DESC SMViewDesc;
SMViewDesc.Format = SMTextureDesc.Format;
SMViewDesc.ViewDimension = D3D11_SRV_DIMENSION_TEXTURECUBE;
SMViewDesc.TextureCube.MipLevels = SMTextureDesc.MipLevels;
SMViewDesc.TextureCube.MostDetailedMip = 0;
result = device->CreateShaderResourceView(SMTexture, &SMViewDesc, &m_texture);
And finally the shaders:
cbuffer MatrixBuffer
{
matrix worldMatrix;
matrix viewMatrix;
matrix projectionMatrix;
};
struct VertexInputType
{
float4 position : POSITION;
float3 tex : TEXCOORD;
float3 normal : NORMAL;
};
struct PixelInputType
{
float4 position : SV_POSITION;
float3 tex : TEXCOORD;
float4 domePosition : TEXCOORD1;
float3 normal : NORMAL;
};
PixelInputType SkyDomeVertexShader(VertexInputType input)
{
PixelInputType output;
input.position.w = 1.0f;
output.position = mul(input.position, worldMatrix);
output.position = mul(output.position, viewMatrix);
output.position = mul(output.position, projectionMatrix);
output.normal = mul(input.normal, (float3x3)worldMatrix);
output.normal = normalize(output.normal);
output.tex = input.tex;
output.domePosition = input.position;
return output;
}
TextureCube cubemap : register(t0);
SamplerState samplerWrap : register(s0);
cbuffer GradientBuffer
{
float4 apexColor;
float4 centerColor;
};
struct PixelInputType
{
float4 position : SV_POSITION;
float3 tex : TEXCOORD;
float4 domePosition : TEXCOORD1;
float3 normal : NORMAL;
};
float4 SkyDomePixelShader(PixelInputType input) : SV_TARGET
{
float height;
float4 outputColor;
outputColor = cubemap.Sample(samplerWrap, input.tex);
return outputColor;
}
Thanks for your help!