I am trying to draw a quad with texturing. The texture should not be pure green (see vector<float> pixel_data).
My source is:
void draw_objects(void)
{
glDisable(GL_LIGHTING);
float projection_modelview_mat[16];
init_perspective_camera(45.0f,
float(win_x) / float(win_y),
0.01f, 10000.0f,
0, 0, 10, // Camera position.
0, 0, 0, // Look at position.
0, 1, 0, // Up direction vector.
projection_modelview_mat);
glActiveTexture(GL_TEXTURE0);
glGenTextures(1, &card_tex);
glBindTexture(GL_TEXTURE_2D, card_tex);
glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST);
glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST);
vector<float> pixel_data;
pixel_data.push_back(0);
pixel_data.push_back(1);
pixel_data.push_back(0);
pixel_data.push_back(1);
pixel_data.push_back(1);
pixel_data.push_back(0);
pixel_data.push_back(0);
pixel_data.push_back(1);
pixel_data.push_back(1);
pixel_data.push_back(1);
pixel_data.push_back(0);
pixel_data.push_back(1);
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB32F, 2, 2,
0, GL_RGB,
GL_FLOAT, &pixel_data[0]);
perspective_tex.use_program();
glUniformMatrix4fv(glGetUniformLocation(perspective_tex.get_program(), "mvp_matrix"), 1, GL_FALSE, &projection_modelview_mat[0]);
glUniform1i(glGetUniformLocation(perspective_tex.get_program(), "tex"), 0);
glGenBuffers(1, &triangle_buffer);
vector<GLfloat> vertex_data = {
// 3D position, 2D texture coordinate
// card front
-1, -1, 0.0, 0, 0, // vertex 0
1, -1, 0.0, 0, 1.0, // vertex 1
1, 1, 0.0, 1.0, 1.0, // vertex 2
-1, -1, 0.0, 0, 0, // vertex 0
1, 1, 0.0, 1.0, 1.0, // vertex 2
-1, 1, 0.0, 1.0, 0, // vertex 3
};
const GLuint components_per_vertex = 5;
const GLuint components_per_position = 3;
const GLuint components_per_tex_coord = 2;
const GLuint num_vertices = vertex_data.size() / components_per_vertex;
glBindBuffer(GL_ARRAY_BUFFER, triangle_buffer);
glBufferData(GL_ARRAY_BUFFER, vertex_data.size() * sizeof(GLfloat), &vertex_data[0], GL_STATIC_DRAW);
glEnableVertexAttribArray(glGetAttribLocation(perspective_tex.get_program(), "position"));
glVertexAttribPointer(glGetAttribLocation(perspective_tex.get_program(), "position"),
components_per_position,
GL_FLOAT,
GL_FALSE,
components_per_vertex * sizeof(GLfloat),
NULL);
glEnableVertexAttribArray(glGetAttribLocation(perspective_tex.get_program(), "tex_coord"));
glVertexAttribPointer(glGetAttribLocation(perspective_tex.get_program(), "tex_coord"),
components_per_tex_coord,
GL_FLOAT,
GL_TRUE,
components_per_vertex * sizeof(GLfloat),
(const GLvoid*)(components_per_position * sizeof(GLfloat)));
glDrawArrays(GL_TRIANGLES, 0, num_vertices);
}
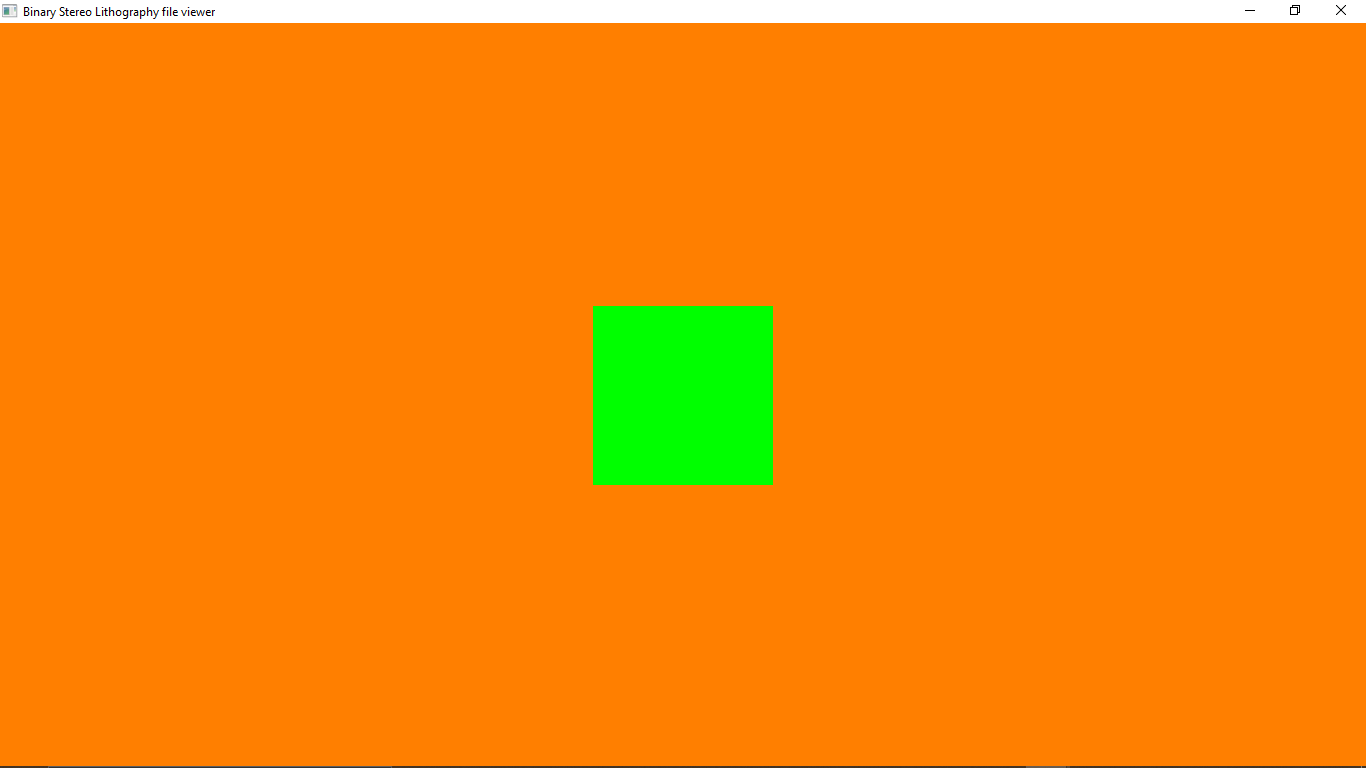