Hello guys,
I hope you're all doing well.
So I created an application on Qt6 that rotates a cube using quaternions. The rotation is achieved with respect to the OpenGL standard coordinate system.
As I know, the OpenGL standard coordinate system is represented in the picture below:
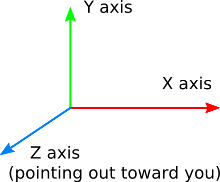
I added a fixed coordinate system to my scene (like the one shown in picture above). So when I insert the quaternion values as follows: w=0.7, x=0.7, y=0.0, z=0.0, the cube rotates around the x axis of the standard coordinate system.
Then I had to change the orientation of that coordinate system's axes as shown in the picture below where:
x is the Magenta axis
y is the White axis
z is the Yellow axis
and my goal is to rotate the cube using quaternion but this time around the new fixed coordinate system, I didn't know how to proceed but this is what I've done so far.
In my paintGL() function, I started by drawing the fixed coordinate system and changing its axes orientation using rotate:
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
//================Orbital Frame ==========================================//
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(2.0,2.0,0.0, 0.0,0.0,-5.0,0.0,1.0,0.0);
glTranslatef(0.0,0.0,-5.0);
glRotatef(180.0,0.0,1.0,0.0);
glRotatef(-90.0,1.0,0.0,0.0);
glScalef(0.4,0.4,0.4);
glMatrixMode(GL_PROJECTION);
DrawOrbitalFrame();
Then, in my program, I have shader programs (Vertex and Fragment shader programs) that I used to draw a textured cube:
program.bind();
texture->bind();
// Calculate model view transformation
QMatrix4x4 localMatrix;
//QMatrix4x4 identity;
localMatrix.lookAt(QVector3D(2.0, 2.0, 0.0), QVector3D(0.0,0.0,-5.0),QVector3D(0.0,1.0,0.0));
localMatrix.translate(0.0, 0.0, -5.0);
localMatrix.scale(0.4,0.4,0.4);
quaternion = QQuaternion(quat_w, quat_x, quat_y, quat_z);
quaternion.normalize(); // Normalizing my quaternion
localMatrix.rotate(quaternion); // the quaternion in here corresponds to the one that can be manually inserted in the spin boxes in the ui
quaternion=csvquaternion;
localMatrix.rotate(quaternion); // the quaternion in here correponds to the one extracted from a QList
program.setUniformValue("mvp_matrix", projection * localMatrix);
update();
// Use texture unit 0 which contains cube.png
program.setUniformValue("texture", 0);
// Draw cube geometry
geometries->drawCubeGeometry(&program);
texture->release();
program.release();
The rotation is achieved using the rotate function but the object is rotating around the axes of the standard coordinate system and not the new one.
Can someone please tell me what can I do to make it rotate around the new fixed coordinate system or give a little tip on how to achieve that ?
Thank you all.