Hi, I am new to game development and I followed a tutorial on a 2d platformer character controller script. Everything is working perfectly, except for the jump height. Jumping while standing still is much lower than jumping while running. I assume somewhere in my code the vector force is being influenced by the horizontal input, but I don't know where. If anyone could help me, I'd greatly appreciate it!
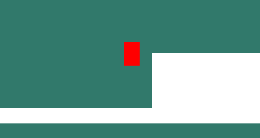

Here is my code:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
[Header("Components")]
private Rigidbody2D rb;
[Header("Layer Masks")]
[SerializeField] private LayerMask groundLayer;
[Header("Movement Variables")]
[SerializeField] private float movementAcceleration;
[SerializeField] private float maxMoveSpeed;
[SerializeField] private float groundLinearDrag;
private float horizontalDirection;
private bool changingDirection => (rb.velocity.x > 0f && horizontalDirection < 0f) || (rb.velocity.x < 0f && horizontalDirection > 0f);
[Header("Jump Variables")]
[SerializeField] private float jumpForce = 12f;
[SerializeField] private float airLinearDrag = 2.5f;
[SerializeField] private float fallMultiplier = 8f;
[SerializeField] private float lowJumpFallMultiplier = 5f;
private bool canJump => Input.GetButtonDown("Jump") && onGround;
[Header("Ground Collision Variables")]
[SerializeField] private float groundRaycastLength = 0.9f;
private bool onGround;
private void Start()
{
rb = GetComponent<Rigidbody2D>();
}
private void Update()
{
horizontalDirection = GetInput().x;
if (canJump) Jump();
}
private void FixedUpdate()
{
CheckCollisions();
MoveCharacter();
if (onGround){
ApplyGroundLinearDrag();
}else{
ApplyAirLinearDrag();
FallMultiplier();
}
}
private Vector2 GetInput()
{
return new Vector2(Input.GetAxisRaw("Horizontal"), Input.GetAxisRaw("Vertical"));
}
private void MoveCharacter()
{
rb.AddForce(new Vector2(horizontalDirection, 0f) * movementAcceleration);
if (Mathf.Abs(rb.velocity.x) > maxMoveSpeed)
rb.velocity = new Vector2(Mathf.Sign(rb.velocity.x) * maxMoveSpeed, rb.velocity.y);
}
private void ApplyGroundLinearDrag()
{
if (Mathf.Abs(horizontalDirection) < 0.4f || changingDirection)
{
rb.drag = groundLinearDrag;
}
else
{
rb.drag = 0f;
}
}
private void ApplyAirLinearDrag()
{
rb.drag = airLinearDrag;
}
private void Jump()
{
ApplyAirLinearDrag();
rb.velocity = new Vector2(rb.velocity.x, 0f);
rb.AddForce(Vector2.up * jumpForce, ForceMode2D.Impulse);
}
private void CheckCollisions()
{
onGround = Physics2D.Raycast(transform.position * groundRaycastLength, Vector2.down, groundRaycastLength, groundLayer);
}
private void OnDrawGizmos(){
Gizmos.color = Color.green;
Gizmos.DrawLine(transform.position, transform.position + Vector3.down * groundRaycastLength);
}
private void FallMultiplier()
{
if (rb.velocity.y < 0)
{
rb.gravityScale = fallMultiplier;
}
else if (rb.velocity.y > 0 && !Input.GetButton("Jump"))
{
rb.gravityScale = lowJumpFallMultiplier;
}
else
{
rb.gravityScale = 1f;
}
}
}