Imagine the following scenario with three hitboxes, before the collision detection & resolution occurs:
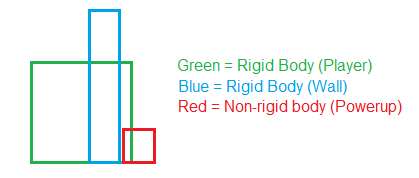
The green rectangle represents the player and is a rigid body. The blue rectangle represents a wall, and is also a rigid body. The red rectangle represents a collectible power-up, which disappears when the player picks it up and is not a rigid body. In this scenario, the player was to the left of the wall, is moving right, and collides with the wall. I've run into a problem where the order that the collision tests between the regions occurs is unpredictable and can result in incorrect behavior.
If the player and the wall collision is resolved first, the player is correctly re-positioned to the left of the wall, and will no longer be colliding with the power-up. However, if the collision test with the power-up is done first, the player would collect it, which is not the correct behavior. Naturally, the scene could also be mirrored, with the power-up to the left of the wall and the player to the right, moving left.
The only thing I can think of to deal with this problem is to do two passes for collection detection, one for collisions between rigid bodies only, and another for non-rigid bodies. This seems really inefficient though, so I'm wondering if there's a better way to address this. Any thoughts would be appreciated. Thanks!