I stole a nice looking shader from ShaderToy in order to run it on my own WebGL2 implementation for learning purposes.
The shader works perfectly on desktop browsers, but when viewing from any phone browser it looks pixelated. I have no idea why though :(
I'll post screenshots and code below, hopefully that can help figure out why this is happening to me. Any suggestions are appreciated! Thanks!
Mobile:
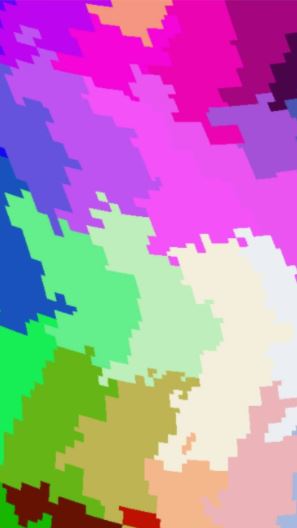
Desktop:
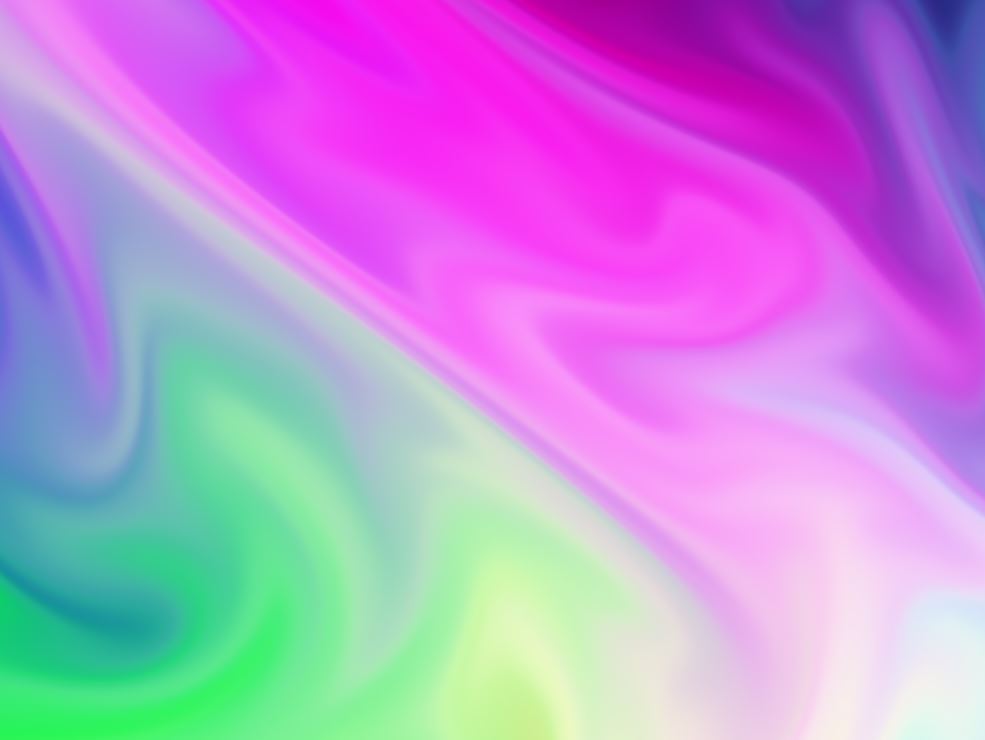
#version 300 es
precision mediump float;
in vec2 vertex;
out vec4 composition;
#define RADIANS 0.017453292519943295
uniform vec2 viewport;
uniform float frameTime;
const int zoom = 40;
const float brightness = 0.975;
float fScale = 1.25;
float cosRange(float degrees, float range, float minimum) {
return (((1.0 + cos(degrees * RADIANS)) * 0.5) * range) + minimum;
}
void testing(vec2 pixel) {
float time = frameTime * 1.25;
vec2 uv = pixel.xy / viewport.xy;
vec2 p = (2.0*pixel.xy-viewport.xy)/max(viewport.x,viewport.y);
float ct = cosRange(time*5.0, 3.0, 1.1);
float xBoost = cosRange(time*0.2, 5.0, 5.0);
float yBoost = cosRange(time*0.1, 10.0, 5.0);
fScale = cosRange(time * 15.5, 1.25, 0.5);
for(int i=1;i<zoom;i++) {
float _i = float(i);
vec2 newp=p;
newp.x+=0.25/_i*sin(_i*p.y+time*cos(ct)*0.5/20.0+0.005*_i)*fScale+xBoost;
newp.y+=0.25/_i*sin(_i*p.x+time*ct*0.3/40.0+0.03*float(i+15))*fScale+yBoost;
p=newp;
}
vec3 col=vec3(0.5*sin(3.0*p.x)+0.5,0.5*sin(3.0*p.y)+0.5,sin(p.x+p.y));
col *= brightness;
// Add border
float vigAmt = 5.0;
float vignette = (1.-vigAmt*(uv.y-.5)*(uv.y-.5))*(1.-vigAmt*(uv.x-.5)*(uv.x-.5));
float extrusion = (col.x + col.y + col.z) / 4.0;
extrusion *= 1.5;
extrusion *= vignette;
composition = vec4(col, 1.0);
// composition = vec4(col, extrusion);
}
void main() {
testing(gl_FragCoord.xy);
}